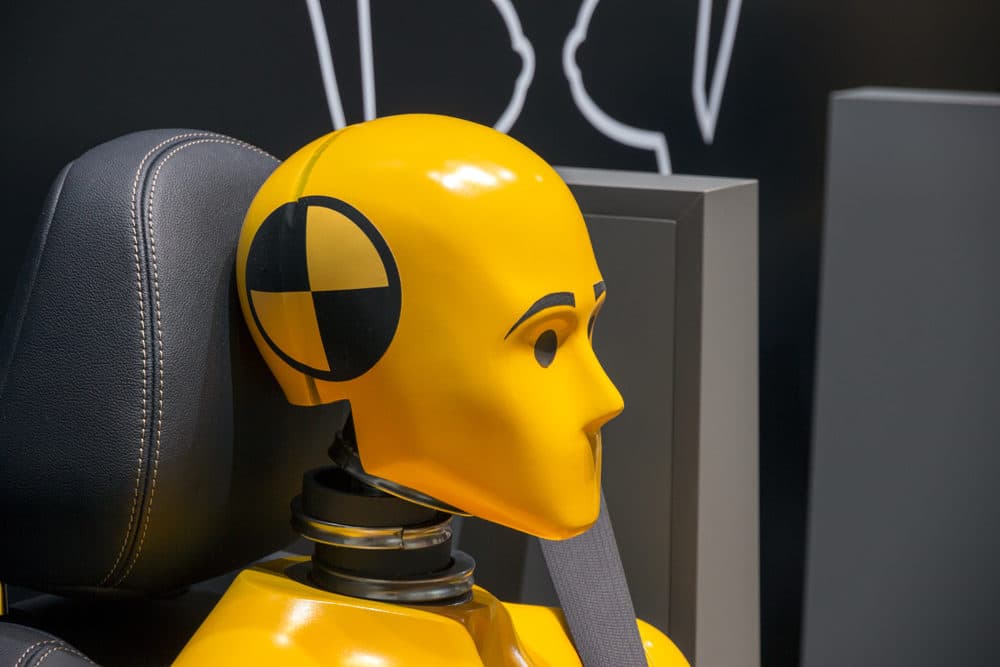
Parameterized testing is a technique that allows you to run the same test multiple times with different input values, as opposed to just repeating the tests with slightly different values. In the testing utilities of the languages that I code, each has means to perform parameterised testing:
Python pytest
import pytest
@pytest.mark.parametrize('name, left, right', [['first', 'a', 'a'],
['second', 'b', 'b'],
['third', 'c', 'c']])
def test_letters_match(name, left, right):
assert left == right, name
JS jest
Note, Usingit.each`table`
with a linter makes life a lot easier. Also, please excuse my code block plugin and how it displays the following example!
test.each`
a | expected
${1.3} | ${1}
${1.7} | ${2}
${2.3} | ${2}
`('Math.round correctly rounds $a', ({a, expected}) => {
expect(Math.round(a)).toBe(expected);
});
PHP PHPUnit
public static function additionProvider(): array
{
return [
[0, 0, 0],
[0, 1, 1],
[1, 0, 1],
[1, 1, 3],
];
}
#[PHPUnit\Framework\Attributes\DataProvider('additionProvider')]
public function testAdd(int $a, int $b, int $expected): void
{
$this->assertSame($expected, $a + $b);
}
Flutter, i.e. Dart
The obvious next question for me was then, how do I achieve this in my Dart code? My IDE wasn't revealing any special methods so I headed over to StackOverflow which yielded this great answer:
Dart'stest
package is smart in that it is not trying to be too clever.
Sometimes, simple is indeed better!
When writing my widget tests, I've opted for the following pattern:
pill_test.dart
void main() {
group('Pill', () {
final styles = <Map>[
{
"name": PillStyle.initial,
"border": hex('#CED0DA'),
"bg": Colors.white,
},
{
"name": PillStyle.warning,
"border": hex('#F7BF53'),
"bg": hex('#FEF8ED'),
},
];
/// .....
for (var styleDef in styles) {
testWidgets(
'colours are correct for ${styleDef["name"]}',
(tester) async {
final widget = Directionality(
textDirection: TextDirection.ltr,
child: Pill(
label: 'Test label',
style: styleDef["name"],
),
);
await tester.pumpWidget(widget);
final dynamic container = tester.widget(find.byType(Container));
expect(container.decoration.color, styleDef['bg']);
expect(
(container.decoration.border as Border).bottom.color,
styleDef['border'],
);
},
);
}
});
}
And that's it!