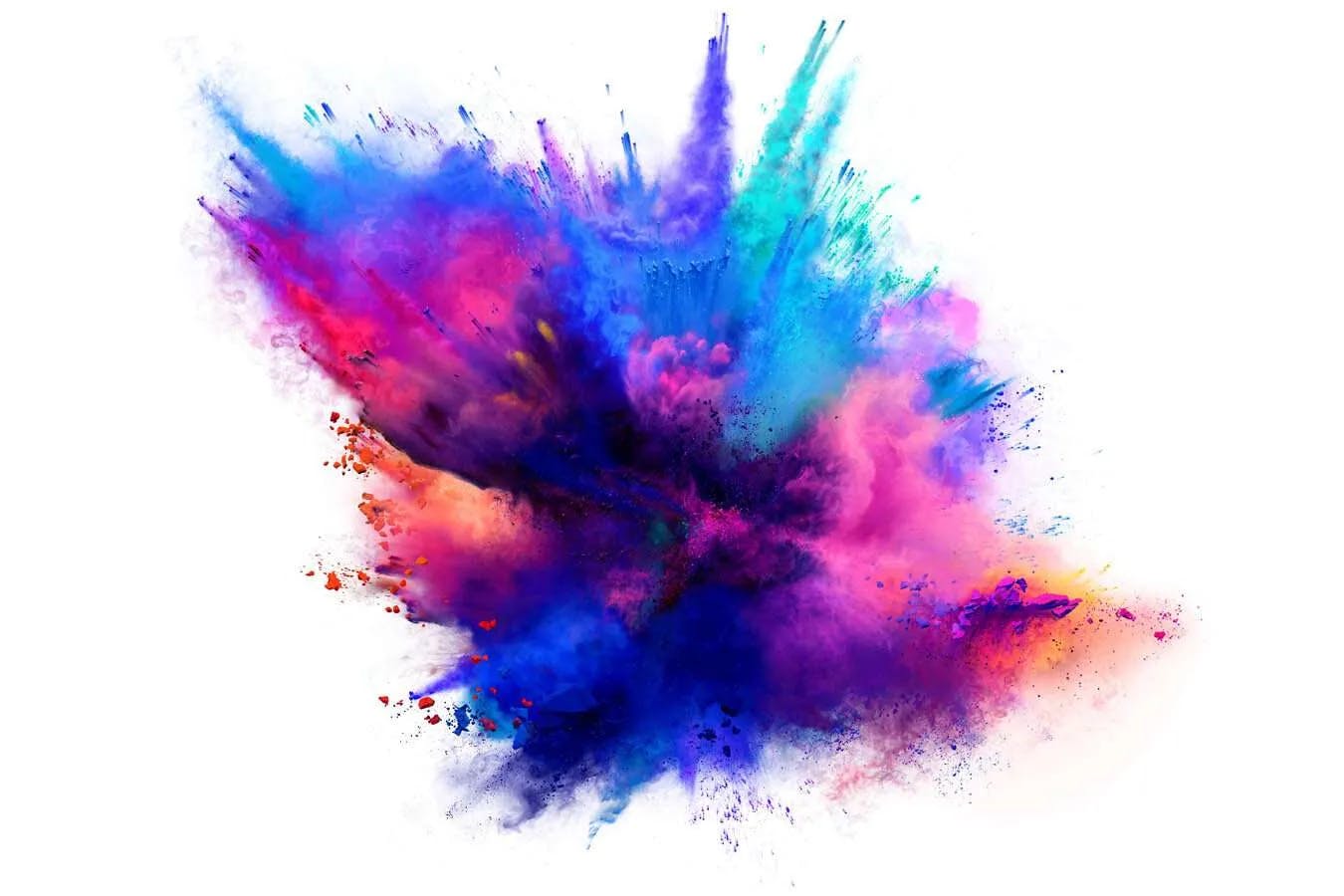
Flutter has a great way of dealing with colours built in which I won't cover here, but if you want to use a "custom" colour, what do you do? Well, it turns out that flutter natively wants to use hexadecimal integers (i.e.
0x...
), as opposed to what I was most used to, i.e. just #FFF
- I didn't like this!I came across this article which inspired a very similar solution:
hex.dart
import 'package:flutter/material.dart';
Color hex(String color) {
color = color.replaceAll("#", "");
switch (color.length) {
case 3:
color = "0xFF$color$color";
break;
case 6:
color = "0xFF$color";
break;
case 8:
color = "0x$color";
default:
throw ArgumentError();
}
return Color(int.parse(color));
}
Test:
hex_test.dart
import 'package:app/helpers/hex.dart';
import 'package:flutter/material.dart';
import 'package:test/test.dart';
void main() {
group('hex()', () {
test('converts a 3-digit hex string to a Color', () {
expect(hex('#fff'), Color(0xFFFFFFFF));
});
test('converts a 6-digit hex string to a Color', () {
expect(hex('#ffffff'), Color(0xFFFFFFFF));
});
test('converts an 8-digit hex string to a Color', () {
expect(hex('#ffff0000'), Color(0xFFFF0000));
});
test('throws an error for an invalid hex string', () {
expect(() => hex('#invalid'), throwsArgumentError);
});
});
}
Usage would then look something like:
Usage
Text(
label,
maxLines: 1,
overflow: TextOverflow.ellipsis,
style: TextStyle(
fontSize: 12,
color: hex('#111637'),
),
)
I don't however know how performant this ends up being. Notice how
TextStyle
can no longer have const
added before it!