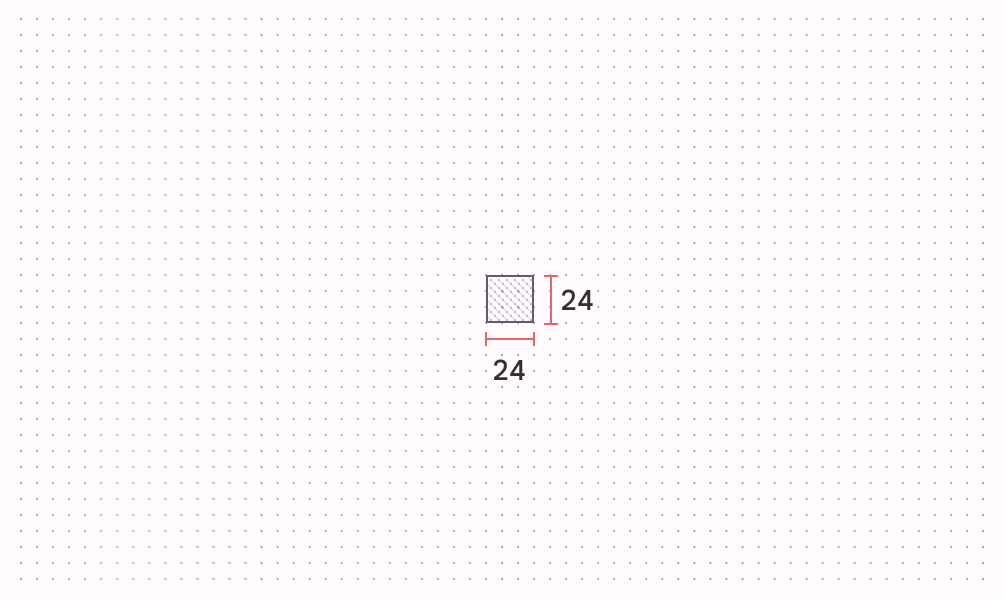
Constraints go down. Sizes go up. Parent sets position.
The notion behind this phrase is key if you're a developer that writes code that effectively generates HTML and CSS. Sizing and spacing is completely different in Flutter and forgetting how sizing works in HTML and CSS is the best way of keeping you enjoying Flutter.
This article from the Flutter docs is mandatory reading in your journey to becoming a layout genius. It will stop a lot of code smell (for which there'll be a lot in your first few components) and once again, keep you enjoying Flutter.
In my Flutter journey, working during the day with React and then the odd evening playing with Flutter, I found switching between the two sizing systems caused me to fill up the swear jar quite a bit. The root cause however, was that I'm kept trying to solve issues with a HTML brain. Don't do that.
There's not much more that I can add compared to what's already in the documentation, so seriously, please read it!
Common issues
I'll add more to this list when I come around to issues
How big is this space?
Don't be tempted to use
MediaQuery.sizeOf(context)
to work out how big a section of your app is, as this command will give you the size of the entire app (obviously this is okay if you really want to know this). What we really need is LayoutBuilder
.import 'package:flutter/material.dart';
class ExampleWidget extends StatelessWidget {
const ExampleWidget({
super.key,
});
@override
Widget build(BuildContext context) {
return LayoutBuilder(
builder: (BuildContext context, BoxConstraints constraints) {
final availableWidth = constraints.maxWidth;
return Text('We have $availableWidth width to use');
},
);
}
}
Ellipsis don't work
Imagine you have the following:
Text(
'Some text',
maxLines: 1,
overflow: TextOverflow.ellipsis,
style: TextStyle(
fontSize: 13,
),
)
One may expect this to just work out of the box, but it won't and this StackOverflow answer explains why: one needs to wrap the widget in a
Flexible
or Expanded
to let your widget know that it's ok for it to be narrower than its intrinsic width.Expanded(
child: Text(
'Some text',
maxLines: 1,
overflow: TextOverflow.ellipsis,
style: TextStyle(
fontSize: 13,
),
),
)
Wrap widget makes everything full width
If you have a content in a
Row
that you want to automatically wrap on to the next line, you can use the Wrap
widget. However, you may find that instead of having everything wrap on to the next line, all of the Wrap
's children
become full width. Inspect your children
to ensure that the Row
has a mainAxisSize
of mainAxisSize.min
.